This simple script will spawn a jet to patrol an AO and attack any enemy vehicle or helicopter it sees. This is improved code that has a proper patrol radius and is better than the jet patrolling in too small a radius.
// Spawn a CAS jet to patrol the AO. #define PLANE_TYPE "rhs_l159_CDF","rhs_l39_cdf","rhs_mig29s_vmf","RHS_Su25SM_vvsc","RHS_T50_vvs_generic","RHS_T50_vvs_generic_ext","RHS_T50_vvs_blueonblue" _plane = createVehicle [([PLANE_TYPE] call BIS_fnc_selectRandom), [0,0,0], [], 0, "FLY"]; _plane addEventHandler ["Fired",{ (_this select 0) setVehicleAmmo 1 }]; _pilotguy = [[0,0,0], EAST, ["rhs_pilot"],[],[],[],[],[],232] call BIS_fnc_spawnGroup; ((units _pilotguy) select 0) moveInDriver _plane; _wpcas = _pilotguy addWaypoint [getmarkerPos "aoMarker", 4500]; _wpcas setWaypointType "LOITER"; _wpcas setWaypointLoiterRadius 4500; _wpcas setWaypointLoiterType "CIRCLE_L"; _wpcas setWaypointBehaviour "AWARE"; _wpcas setWaypointCombatMode "RED"; |
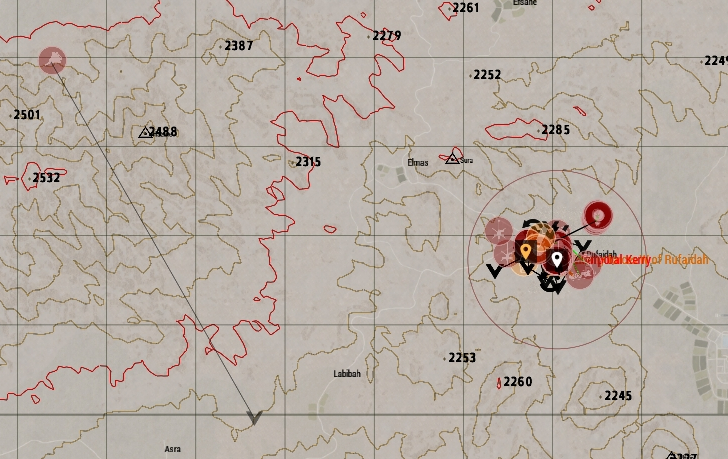
This spawns the jet in the air at the Molos airfield and it will move to the marker named mkr1 and patrol this area. Very useful indeed. Put this script into the initServer.sqf and this will work in your mission.
Use the code below to spawn an OPFOR tank platoon to patrol an AO. They will spawn at the salt flats and will then move to the object named man1.
_randTanks90 = [[23762.7,18920.1,3.33786e-006] , 0, 400, 10, 0, 0.3, 0] call BIS_fnc_findSafePos; _newtanks29 = [_randTanks90, EAST, (configFile >> "CfgGroups" >> "EAST" >> "OPF_F" >> "Armored" >> "OIA_TankPlatoon")] call BIS_fnc_spawnGroup; [_newtanks29, man1 getRelPos [15, 0], 600] call bis_fnc_taskPatrol; |
Finally, this code will spawn an OPFOR Kajman that will fly to the HQ and then the crew will get out and shut down the chopper. The chopper will spawn at the onbject named heli99 and will move to the object named man1, then they will land and exit the vehicle.
_randPos11 = [getPos heli99 , 0, 1200, 12, 0, 0.3, 0] call BIS_fnc_findSafePos; _heli1 = [_randPos11, 0, "O_Heli_Attack_02_F", EAST] call BIS_fnc_spawnVehicle; _group_ifrit1 = _heli1 select 2; _wp = _group_ifrit1 addWaypoint [getPosATL man1, 0]; _wp setWaypointBehaviour "SAFE"; _wp setWaypointCombatMode "GREEN"; _wp setWaypointCompletionRadius 1300; _wp setWaypointSpeed "FULL"; _wp setwaypointType "GETOUT"; _group_ifrit1 = _heli1 select 2; _wp2 = _group_ifrit1 addWaypoint [getPosATL man1, 0]; _wp2 setWaypointBehaviour "SAFE"; _wp2 setWaypointCombatMode "GREEN"; _wp2 setWaypointCompletionRadius 1300; _wp2 setWaypointSpeed "FULL"; _wp2 setwaypointType "DISMISSED"; |
Complete code to spawn a mission with a radio tower and a HQ. You just need a huge marker covering most of Altis named mkr1.
// Find a random area to spawn the HQ. This is within the area of the marker "mkr1". _mrk = "mkr1"; _area = markerSize _mrk; _nul = _area pushBack markerDir _mrk; _nul = _area pushBack ( markerShape _mrk isEqualTo "Rectangle" ); _pos = [ _mrk, _area ] call BIS_fnc_randomPosTrigger; _randPos = [_pos , 0, 1200, 12, 0, 0.3, 0] call BIS_fnc_findSafePos; _cargo = "Land_Cargo_HQ_V1_F" createVehicle _randPos; _randPos14 = _cargo getRelPos [9, 12]; _mg13 = createVehicle ["O_GMG_01_high_F", _randPos14, [], 0, "CAN_COLLIDE"]; _mgguy13 = [_randPos14, EAST, ["O_Soldier_F"],[],[],[],[],[],232] call BIS_fnc_spawnGroup; ((units _mgguy13) select 0) moveInGunner _mg13; // Spawning the radio tower. _randPos2 = [_pos , 0, 1200, 10, 0, 0.3, 0] call BIS_fnc_findSafePos; _tower = "Land_TTowerBig_1_F" createVehicle _randPos2; _tower setVectorUp [0,0,1]; // Make sure the tower is not leaning. _towerbox = "Land_spp_Transformer_F" createVehicle _randPos2; _towerbox setVectorUp [0,0,1]; _towerbox2 = "Land_TTowerSmall_1_F" createVehicle _randPos2; _towerbox2 setVectorUp [0,0,1]; _tower setVehicleVarName "tower1"; tower1 = _tower; _genny = _tower getRelPos [7, 9]; // Spawn a random minefield around the radio tower. // ********************************************************************* _initialPos = getPos tower1; // Pos of tower. for "_count" from 1 to 50 do { _minePos = [ (_initialPos select 0) + ((random 80) - 40), (_initialPos select 1) + ((random 80) - 40) ]; _minen = createMine [ "APERSBoundingMine", _minePos, [], 0 ]; }; // End mine code. // ********************************************************************* _minefield = "Land_PowerGenerator_F" createVehicle _genny; // Spawning a MG guy near tower. _randPos3 = [_randPos2, 1, 60, 3, 0, 20, 0] call BIS_fnc_findSafePos; _mg = createVehicle ["O_HMG_01_high_F", _randPos3, [], 0, "CAN_COLLIDE"]; _mgguy = [_randPos3, EAST, ["O_Soldier_F"],[],[],[],[],[],232] call BIS_fnc_spawnGroup; ((units _mgguy) select 0) moveInGunner _mg; // Spawning a couple of infantry groups to defend the HQ. // ********************************************************************* _hqdef2 = _cargo getRelPos [-96, 256]; _group = [_hqdef2, EAST, configfile >> "CfgGroups" >> "East" >> "OPF_F" >> "Infantry" >> "OIA_InfSquad_Weapons"] call BIS_fnc_spawnGroup; private _buildingPositions = _cargo buildingPos -1; private _buildingPosition = [0,0,0]; if (!(_buildingPositions isEqualTo [])) then { { if (_buildingPositions isEqualTo []) exitWith {}; _buildingPosition = selectRandom _buildingPositions; _x setPos _buildingPosition; _x disableAI 'PATH'; _buildingPositions deleteAt (_buildingPositions find _buildingPosition); } forEach (units _group); }; _randPos99 = [_pos , 0, 1200, 12, 0, 0.3, 0] call BIS_fnc_findSafePos; _towerhq = "CamoNet_OPFOR_big_Curator_F" createVehicle _randPos99; _towerSTUFF = "Box_East_Grenades_F" createVehicle _randPos99; _ammo1 = _towerSTUFF getRelPos [2, 2]; box1 = "O_supplyCrate_F" createVehicle _ammo1; _ammo2 = _towerSTUFF getRelPos [-2, 2]; box2 = "Box_East_WpsLaunch_F" createVehicle _ammo1; _ammo3 = _towerSTUFF getRelPos [3, 2]; box3 = "Box_East_Ammo_F" createVehicle _ammo1; _ammo4 = _towerSTUFF getRelPos [3, 4]; box4 = "Box_East_Wps_F" createVehicle _ammo1; _hqdef3 = _towerhq getRelPos [16, 23]; _group99 = [_hqdef3, EAST, configfile >> "CfgGroups" >> "East" >> "OPF_T_F" >> "Infantry" >> "OIA_InfSquad_Weapons"] call BIS_fnc_spawnGroup; [_group99, getPos _towerhq, 300] call bis_fnc_taskPatrol; _officer = _group createUnit ["O_officer_F",_randPos3,[],0.6,"Colonel"]; _officer setVehicleVarName "man1"; man1 = _officer; man1 setpos (_cargo buildingpos 1); _officer disableAI 'PATH'; // ********************************************************************* //Spawn the group private "_group2"; _group2 = [_randPos3, EAST, configfile >> "CfgGroups" >> "East" >> "OPF_T_F" >> "Infantry" >> "OIA_InfSquad_Weapons"] call BIS_fnc_spawnGroup; //Make the group defend the area. [_group2, _randPos] call bis_fnc_taskDefend; |
More useful Arma 3 script samples: http://securitronlinux.com/battlefield/some-more-arma-3-script-samples/.